Index
このセクションでは前回のセクションのコードを書き替えて複数のブロックを描画します。
ブロック変数の更新
ブロックの基準点の座標bx
、by
を配列bx_list
、by_list
に変更して値を代入し、横のブロック同士の距離をbd
で初期化します。
// ブロック変数
let bx_list = [0, 200, 400, 600];
let by_list = [100, 125, 150, 200];
let bw = 50;
let bs = 140;
let bd = 200;
JavaScript// ブロック変数
let bx_list = [0, 200, 400, 600];
let by_list = [100, 125, 150, 200];
let bw = 50;
let bs = 140;
let bd = 200;
JavaScriptdraw_blocks関数の更新
bx_list
とby_list
、for
文を使用して、draw_blocks
関数を書き換えます。
// ブロックの描画
function draw_blocks() {
ctx.strokeStyle = BLOCK_STROCK_COLOR;
ctx.fillStyle = BLOCK_COLOR;
for (let i = 0; i < bx_list.length; i++) {
// 上ブロック
ctx.strokeRect(bx_list[i] - bw / 2, 0, bw, by_list[i] - bs / 2);
ctx.fillRect(bx_list[i] - bw / 2, 0, bw, by_list[i] - bs / 2);
// 下ブロック
ctx.strokeRect(bx_list[i] - bw / 2, by_list[i] + bs / 2, bw, cvs.height - (by_list[i] + bs / 2));
ctx.fillRect(bx_list[i] - bw / 2, by_list[i] + bs / 2, bw, cvs.height - (by_list[i] + bs / 2));
}
}
JavaScript// ブロックの描画
function draw_blocks() {
ctx.strokeStyle = BLOCK_STROCK_COLOR;
ctx.fillStyle = BLOCK_COLOR;
for (let i = 0; i < bx_list.length; i++) {
// 上ブロック
ctx.strokeRect(bx_list[i] - bw / 2, 0, bw, by_list[i] - bs / 2);
ctx.fillRect(bx_list[i] - bw / 2, 0, bw, by_list[i] - bs / 2);
// 下ブロック
ctx.strokeRect(bx_list[i] - bw / 2, by_list[i] + bs / 2, bw, cvs.height - (by_list[i] + bs / 2));
ctx.fillRect(bx_list[i] - bw / 2, by_list[i] + bs / 2, bw, cvs.height - (by_list[i] + bs / 2));
}
}
JavaScriptfor
文の処理を確認します。for
文で使用する変数i
は0
で初期化して、bx_list
の長さ分ループさせて、ループするたびにi
を1
ずつ大きくします。また、strokeRect()
、fillRect()
のbx
をbx_list[i]
に、by
をby_list[i]
に変更します。変数bd
は次のセクションで使用します。
以上でブロックの描画設定が終わりました。最終的にmain.js
は以下のようになります。
// 描画コンテキストの取得
let cvs = document.querySelector("canvas");
let ctx = cvs.getContext("2d");
// 色
const BACKGROUND_COLOR = "hsl(200deg, 100%, 70%)";
const PLAYER_COLOR = "hsl(0deg, 100%, 60%)";
const PLAYER_STROKE_COLOR = "hsl(0deg, 0%, 100%)";
const BLOCK_COLOR = "hsl(230deg, 100%, 70%)";
const BLOCK_STROCK_COLOR = "hsl(0deg, 0%, 0%)";
// ゲーム変数
const FRAME_ELEMENT = 1000 / 30;
// キャンバス変数
let line_width = 2;
// プレイヤー変数
// 座標
let px = 70;
let py = 50;
// サイズ
let ps = 20;
// 速度
let pvy = 8;
// 基準速度
let standard_pvy = 8;
// ジャンプした場合の速度
let jump_pvy = -15;
// ジャンプできる最低速度
let min_jump_pvy = 6;
// 基準速度未満の場合の追加速度
let add_pvy = 1.5;
// ジャンプ判定
let jump_flag = false;
// ブロック変数
let bx_list = [0, 200, 400, 600];
let by_list = [100, 125, 150, 200];
let bw = 50;
let bs = 140;
let bd = 200;
// キーイベント
document.addEventListener("keydown", event => {
if (event.key === " ") {
if (pvy >= min_jump_pvy) {
jump_flag = true;
}
}
});
// 初期化処理
function init() {
ctx.lineWidth = line_width;
}
// プレイヤーの更新
function update_player() {
// ジャンプ時の処理
if (jump_flag) {
jump_flag = false;
pvy = jump_pvy;
}
// 基準速度未満であれば追加速度を与える
if (pvy < standard_pvy) {
pvy += add_pvy;
}
// 基準速度を超えていれば基準速度にする
if (pvy > standard_pvy) {
pvy = standard_pvy;
}
// 速度をもとに座標を変更
py += pvy;
}
// 背景の描画
function draw_background() {
ctx.fillStyle = BACKGROUND_COLOR;
ctx.fillRect(0, 0, cvs.width, cvs.height);
}
// プレイヤーの描画
function draw_player() {
ctx.strokeStyle = PLAYER_STROKE_COLOR;
ctx.fillStyle = PLAYER_COLOR;
ctx.strokeRect(px - ps / 2, py - ps / 2, ps, ps);
ctx.fillRect(px - ps / 2, py - ps / 2, ps, ps);
}
// ブロックの描画
function draw_blocks() {
ctx.strokeStyle = BLOCK_STROCK_COLOR;
ctx.fillStyle = BLOCK_COLOR;
for (let i = 0; i < bx_list.length; i++) {
// 上ブロック
ctx.strokeRect(bx_list[i] - bw / 2, 0, bw, by_list[i] - bs / 2);
ctx.fillRect(bx_list[i] - bw / 2, 0, bw, by_list[i] - bs / 2);
// 下ブロック
ctx.strokeRect(bx_list[i] - bw / 2, by_list[i] + bs / 2, bw, cvs.height - (by_list[i] + bs / 2));
ctx.fillRect(bx_list[i] - bw / 2, by_list[i] + bs / 2, bw, cvs.height - (by_list[i] + bs / 2));
}
}
function main() {
update_player();
draw_background();
draw_blocks();
draw_player();
setTimeout(main, FRAME_ELEMENT);
}
init();
main();
JavaScript// 描画コンテキストの取得
let cvs = document.querySelector("canvas");
let ctx = cvs.getContext("2d");
// 色
const BACKGROUND_COLOR = "hsl(200deg, 100%, 70%)";
const PLAYER_COLOR = "hsl(0deg, 100%, 60%)";
const PLAYER_STROKE_COLOR = "hsl(0deg, 0%, 100%)";
const BLOCK_COLOR = "hsl(230deg, 100%, 70%)";
const BLOCK_STROCK_COLOR = "hsl(0deg, 0%, 0%)";
// ゲーム変数
const FRAME_ELEMENT = 1000 / 30;
// キャンバス変数
let line_width = 2;
// プレイヤー変数
// 座標
let px = 70;
let py = 50;
// サイズ
let ps = 20;
// 速度
let pvy = 8;
// 基準速度
let standard_pvy = 8;
// ジャンプした場合の速度
let jump_pvy = -15;
// ジャンプできる最低速度
let min_jump_pvy = 6;
// 基準速度未満の場合の追加速度
let add_pvy = 1.5;
// ジャンプ判定
let jump_flag = false;
// ブロック変数
let bx_list = [0, 200, 400, 600];
let by_list = [100, 125, 150, 200];
let bw = 50;
let bs = 140;
let bd = 200;
// キーイベント
document.addEventListener("keydown", event => {
if (event.key === " ") {
if (pvy >= min_jump_pvy) {
jump_flag = true;
}
}
});
// 初期化処理
function init() {
ctx.lineWidth = line_width;
}
// プレイヤーの更新
function update_player() {
// ジャンプ時の処理
if (jump_flag) {
jump_flag = false;
pvy = jump_pvy;
}
// 基準速度未満であれば追加速度を与える
if (pvy < standard_pvy) {
pvy += add_pvy;
}
// 基準速度を超えていれば基準速度にする
if (pvy > standard_pvy) {
pvy = standard_pvy;
}
// 速度をもとに座標を変更
py += pvy;
}
// 背景の描画
function draw_background() {
ctx.fillStyle = BACKGROUND_COLOR;
ctx.fillRect(0, 0, cvs.width, cvs.height);
}
// プレイヤーの描画
function draw_player() {
ctx.strokeStyle = PLAYER_STROKE_COLOR;
ctx.fillStyle = PLAYER_COLOR;
ctx.strokeRect(px - ps / 2, py - ps / 2, ps, ps);
ctx.fillRect(px - ps / 2, py - ps / 2, ps, ps);
}
// ブロックの描画
function draw_blocks() {
ctx.strokeStyle = BLOCK_STROCK_COLOR;
ctx.fillStyle = BLOCK_COLOR;
for (let i = 0; i < bx_list.length; i++) {
// 上ブロック
ctx.strokeRect(bx_list[i] - bw / 2, 0, bw, by_list[i] - bs / 2);
ctx.fillRect(bx_list[i] - bw / 2, 0, bw, by_list[i] - bs / 2);
// 下ブロック
ctx.strokeRect(bx_list[i] - bw / 2, by_list[i] + bs / 2, bw, cvs.height - (by_list[i] + bs / 2));
ctx.fillRect(bx_list[i] - bw / 2, by_list[i] + bs / 2, bw, cvs.height - (by_list[i] + bs / 2));
}
}
function main() {
update_player();
draw_background();
draw_blocks();
draw_player();
setTimeout(main, FRAME_ELEMENT);
}
init();
main();
JavaScript実行結果
Chrome
に移動してindex.html
を更新します。実行結果は以下の画像のようになります。4つ目のブロック対はキャンバスの外にあるため見えませんが、複数のブロック対を描画することができました。
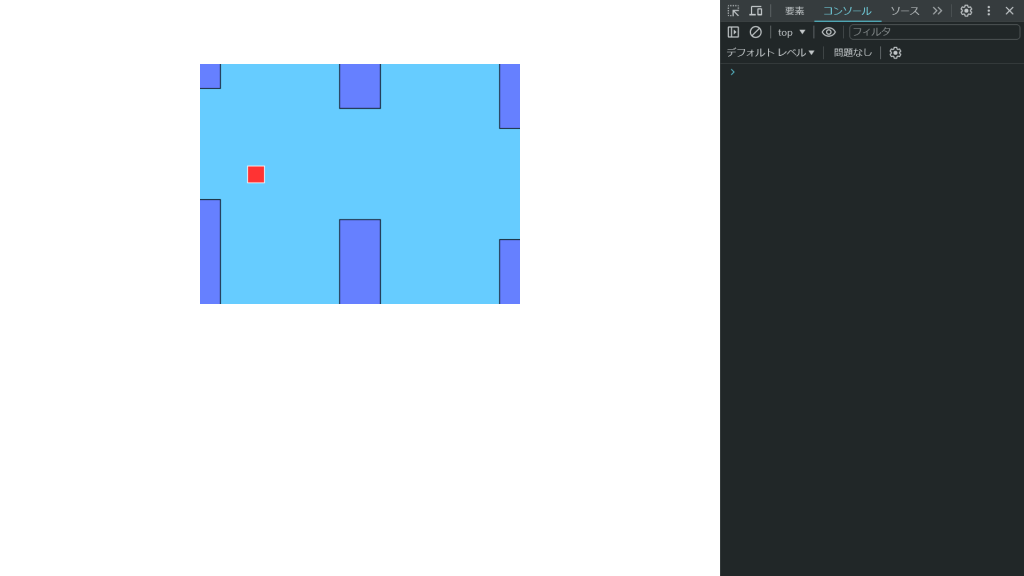
コメント
コメントはまだありません。